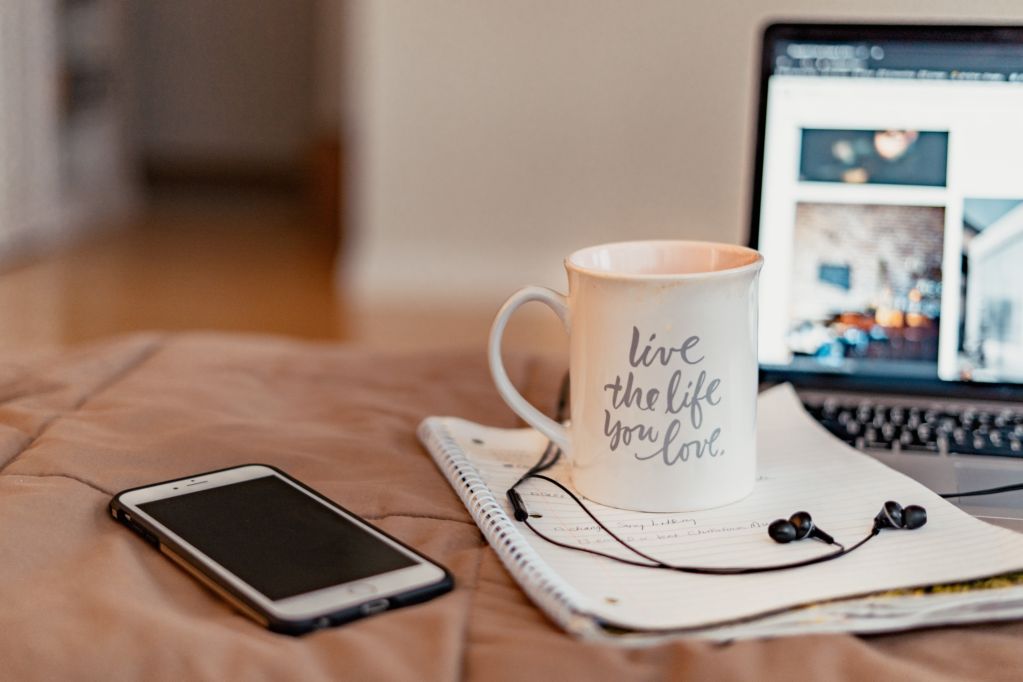
Data Structure
Introduction: Basic Terminology; Elementary Data Organization; Data Structures; Data
Structure Operations; Control Structures; Algorithms: Complexity, Time-Space Tradeoff,
Mathematical Notation and function, String Processing: String Operations, word processing,
and Pattern Matching Algorithms.
Arrays, Records and Pointers: Linear Arrays; Representation of linear array in memory;
Traversing linear arrays, Inserting and Deleting; Sorting; (Bubble sort), Searching (linear, binary),
Multidimensional Arrays; Pointer Arrays; Record Structures; Matrices.
Linked lists: Representation of Linked lists in memory, Traversing a linked list, Searching a
linked list, insertion, deletion; Header and two-way lists.
Stacks, Queues, Recursion: Array Representation of Stacks, Polish Notation; Quicksort,
Recursive definition; Towers of Hanoi, Implementation of Recursive procedures, Queue
Dequeue, Priority Queues.
Trees: Binary Trees; Representing Binary Trees in memory, traversing binary tree, Header
Nodes; Threads , binary search trees, Heap tree, heap sort, Huffman’s Algorithm.
Graphs: Sequential Representation of Graph; Adjacency Matrix; Path Matrix; Warshall’s
Algorithm; Linked representation of Graphs.
Responsible | Aishwarza Panday |
---|---|
Last Update | 10/30/2022 |
Completion Time | 21 hours 55 minutes |
Members | 3 |
-
Introduction And Overview
-
Lecture1
-
LECTURE 2 DS
-
Control Structure
-
Practical_task2
-
-
Preliminaries
-
DS Manual 3
-
Preliminaries
-
-
Arrays ,Record and Pointers
-
Arrays and Records.pptx
-
Bubble Sort.pptx
-
binary search.pptx
-
Code implementation of searching and sorting.docx
-
-
Quick Sort
-
QUICK SORT in c++.pptx
-
Quick sort using right as pivot.docx
-
Code implementation of Quick Sort
-
-
Linked List
-
Linked List in c++
-
-
STACK,QUEUE,RECURSION
-
stack & queue code.docx
-
STACK.pptx
-
-
Graph
-
Graph.pptx
-
Floyd-Warshall Algorithm.
-
-
Introduction to tree
-
Huffman Coding Algorithm.pptx
-
Heap Data Structures
-
Tree Traversing
-